You will start by defining the parameters as this is the first section in our template. If needed, you can define the parameters in a separate file that can be referenced when you run the template. In this case, you will be writing the parameters in your template file itself.
If you navigate to the parameters section and press N in Visual Studio Code, you will see the code snippet to add a new parameter, as shown in Figure 8.9.
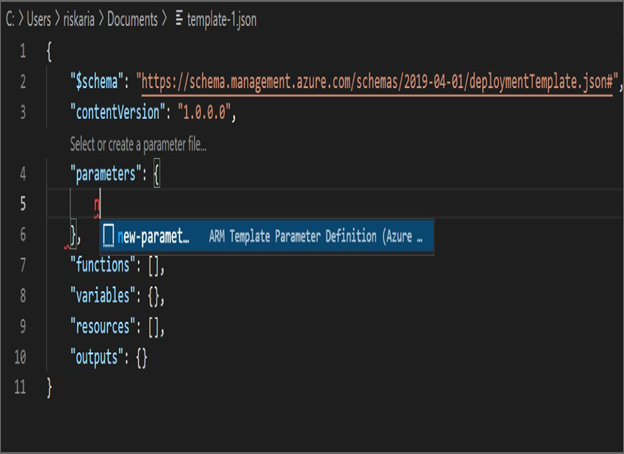
FIGURE 8.9 Adding a new parameter
You can select the code snippet and Visual Studio will immediately generate the code snippet for a new parameter as shown in Figure 8.10.
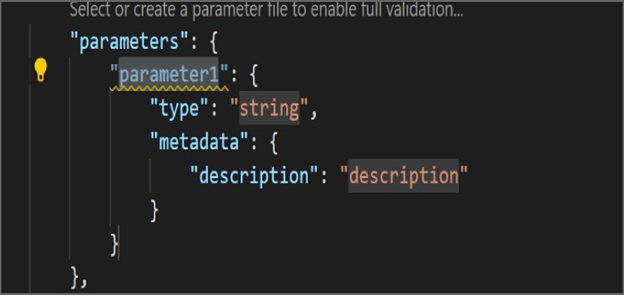
FIGURE 8.10 New parameter code block
Per the requirements, you need the following parameters:
- nameParam: This has a minimum length of 3 and maximum length of 10.
- location: The default value is the location of the resource group. This can be pulled using [resourceGroup().location].
- accountType: The default value is Standard_LRS. Allowed values include Standard_GRS and Standard_ZRS.
The following is the parameter list with the requirements incorporated:
“parameters”:{
“nameParam”: {
“type”: ”string”,
“minLength”: 3,
“maxLength”: 10,
“metadata”: {
“description”: ”User input - name”
}
},
“location”: {
“type”: ”string”,
“defaultValue”: ”[resourceGroup().location]”,
“metadata”: {
“description”: ”Location of the resource”
}
},
“accountType”: {
“type”: ”string”,
“defaultValue” : ”Standard_LRS”,
“allowedValues” : [
“Standard_LRS”,
“Standard_GRS”,
“Standard_ZRS”
],
“metadata”: {
“description”: ”Account type”
}
}
},
As you can see, you are using minLength, maxLength, allowedValues, and defaultValue to define the parameters per your requirements. Now that you have your parameters ready, let’s declare our variable storageAccountName.
Defining Variables
You will be using the variables section in your template to define your variables. Per the requirements, you need to append the nameParam parameter and a string datastore to declare a variable called storageAccountName. You will be using the concat() option to concatenate the name parameter and the string. Here you can also leverage the code snippets in Visual Studio Code. In the following code, you can see that we are appending nameParam and a string and then storing the value to the variable:
“variables”:{
“storageAccountName”: ”[concat(parameters(‘nameParam’),’datastore’)]”
},
Since you declared your variable, let’s go ahead and see how you can create your resource referencing the parameters and variables.
Defining Resources
The resources section is an array; you can add multiple resources that need to be deployed in this section. In this case, you only need a storage account, and you will make use of the code snippet to generate the code block. In the resources section, you can start typing storage, and you will see azure-storage, as shown in Figure 8.11.
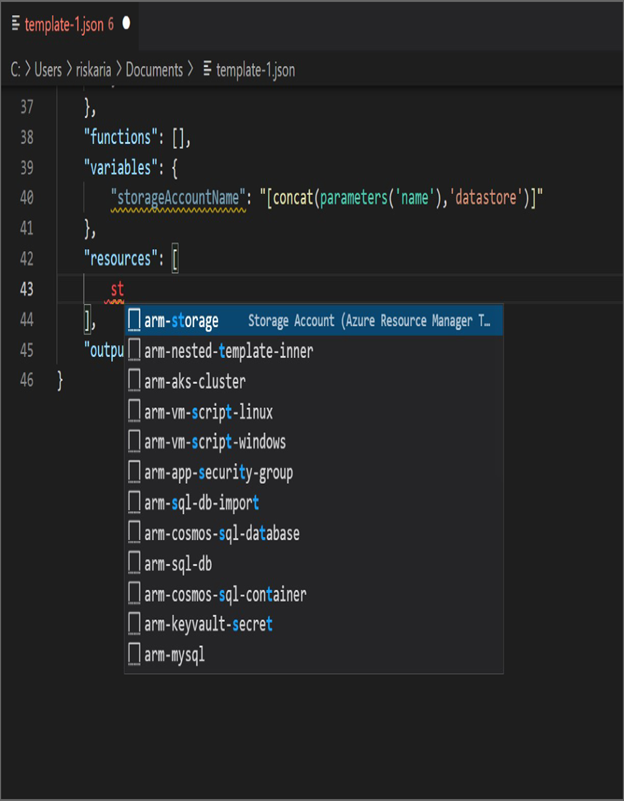
FIGURE 8.11 Adding resource
Now you can remove the default values and give references to your parameters and variables. The final code will look similar to the following:
“resources”:[
{
“name”: ”[variables(‘storageAccountName’)]”,
“type”: ”Microsoft.Storage/storageAccounts”,
“apiVersion”: ”2021-04-01″,
“location”: ”[parameters(‘location’)]”
“kind”: ”StorageV2″,
“sku”: {
“name”: ”[parameters(‘accountType’)]”,
“tier”: ”Standard”
}
}
]
You can remove tags and other options that are not mandatory. The only thing remaining is to display the storage endpoints once the deployment is done. Let’s define that under outputs.