You can compose templates in any text editor you like. However, Visual Studio Code offers the best IntelliSense and code snippets. Once the template is composed, you will be deploying that to a resource group using the Azure portal, Azure PowerShell, or the Azure CLI. In Azure PowerShell, you will be using the New-AzResourceGroupDeployment command by specifying the ARM template using the TemplateUri or TemplateFile parameter. You can use TemplateUri if you have the template file stored in some shared location or source control, and you can use TemplateFile if you composed the file locally. Similarly, in the Azure CLI, you will be using az group deployment create and specify the template location using –template-file or –template-uri. In both Azure PowerShell and the Azure CLI, you need to give a name for the deployment to track it and the targeted resource group name.
Let’s write our first ARM template and deploy it to our Azure subscription in Exercise 8.1.
EXERCISE 8.1
Composing an ARM Template
- In Visual Studio Code, open a new file by pressing Ctrl+N or selecting File ➢ New File.
- Select Azure Resource Manager Template as the language, as you saw in Figure 8.5.
- Type arm, and the code snippets will start showing up; if not, after typing hit Ctrl+spacebar. From the suggestions, select arm!, which is the code snippet for a resource group deployment.
- The skeleton code with all the sections will be populated on your screen. Now without making any changes, let’s deploy this as a template.
- From the Visual Studio Code toolbar, select Terminal ➢ New Terminal. This will open a new PowerShell terminal toward the bottom of the screen.
- Here you need to make sure that the Azure PowerShell module is installed on the computer you are trying this on. You can download the Azure PowerShell module from here:
https://docs.microsoft.com/en-us/powershell/azure/install-az-ps?view=azps-6.5.0
- Log in to your Azure account using the Login-AzAccount command. A sign-in window will pop up, and you can complete the sign-in.
- Once you are signed in, create a new resource group using the New-AzResourceGroup command, for example, New-AzResourceGroup -Name -Location .
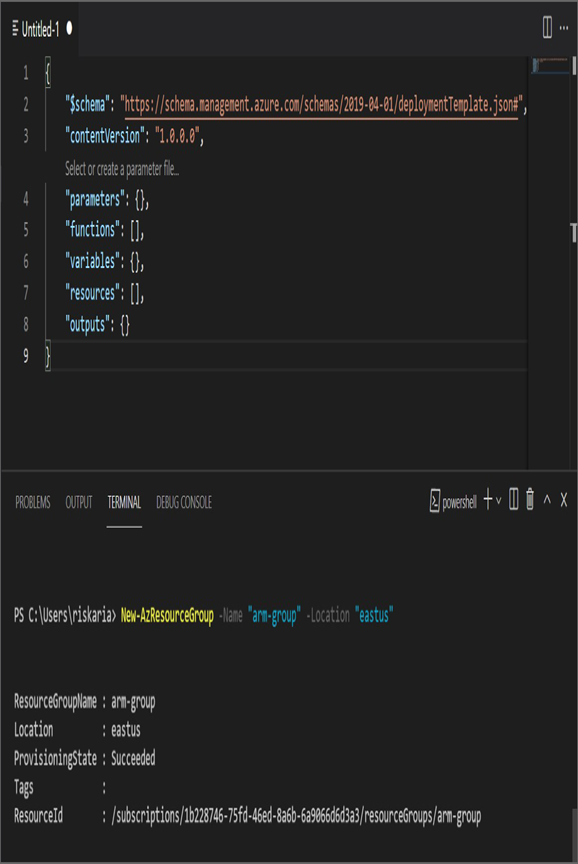
- Save the file in JSON format by hitting Ctrl+S, and now that you have the target resource group, let’s push the template to the resource group using the New-AzResourceGroupDeployment command. The mandatory parameters include the name of the deployment, resource group, and path to the template file, for example: New-AzResourceGroupDeployment -Name “deployment-1” -ResourceGroupName “arm-group” -TemplateFile .\Documents\template-1.json.
- If the deployment is successful, you will see a succeeded message in the terminal.
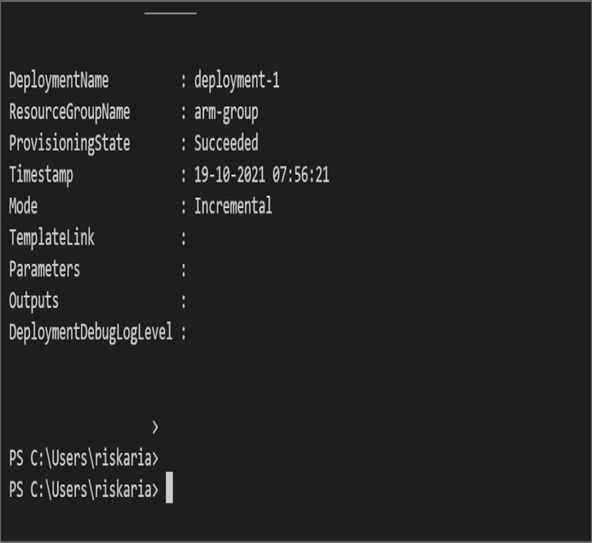
- Sign in to the Azure portal and navigate to the resource group that you created in step 8. There won’t be any resources as our resources section was empty and didn’t instruct ARM to deploy any resources.
- If you navigate to the Deployments blade, you will be able to see the deployment-1 you made. Clicking the deployment name will show you the overview, inputs, outputs, and the template used for the deployment.
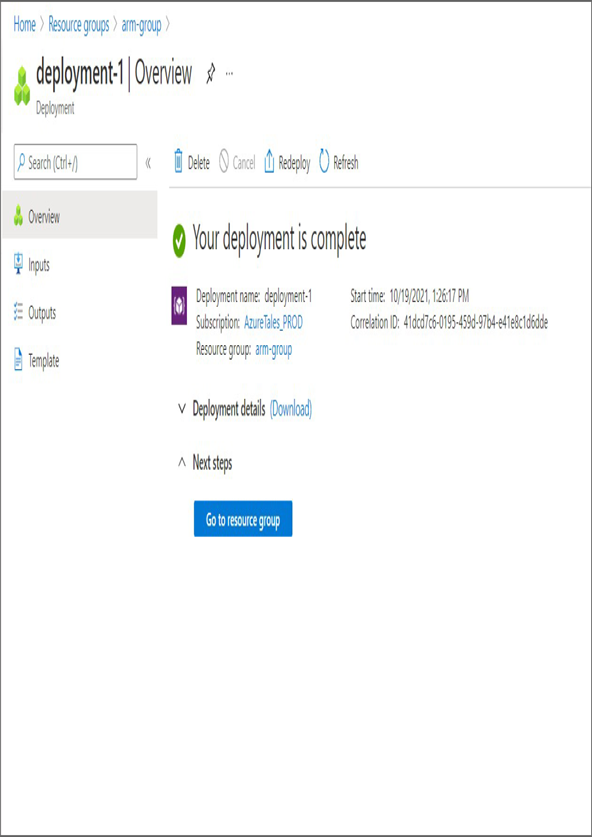
In Exercise 8.1, you saw how to deploy using the ARM templates. Now you will start writing a template that is a bit more advanced. Though this is not marked as an exercise, you can consider this an exercise and build this template by following the instructions. Let’s start by creating the code snippet of the resource group deployment from Visual Studio Code. Once you have the skeleton, you will skip the schema and content version and start defining the parameters first.
In this example, you will be creating a storage account. Let’s assume that your organization creates storage accounts in a frequent manner for your application needs. You need a template where the user will input a keyword and a storage account is created based on the following requirements:
- The template should take a parameter called nameParam that should be of minimum length 3 and maximum length 10.
- The template should take a parameter called location. The default value for this should be the location of the resource group.
- You need a variable called storageAccountName that is constructed by appending the name parameter to the string datastore.
- You need a parameter called accountType. The default value is Standard_LRS. Other supported values include Standard_GRS and Standard_ZRS.
- After deployment, the storage endpoints should be shown to the end user.
Let’s see how you can build a template based on the requirements.